Uncomment missing debug implementations rule
This commit is contained in:
parent
42e775fece
commit
d136b7ce64
@ -1,11 +1,11 @@
|
||||
//! The core library of [Iced].
|
||||
//!
|
||||
//! 
|
||||
//!
|
||||
//! This library holds basic types that can be reused and re-exported in
|
||||
//! different runtime implementations. For instance, both [`iced_native`] and
|
||||
//! [`iced_web`] are built on top of `iced_core`.
|
||||
//!
|
||||
//! 
|
||||
//!
|
||||
//! [Iced]: https://github.com/hecrj/iced
|
||||
//! [`iced_native`]: https://github.com/hecrj/iced/tree/master/native
|
||||
//! [`iced_web`]: https://github.com/hecrj/iced/tree/master/web
|
||||
|
@ -13,6 +13,7 @@ use crate::{
|
||||
/// [built-in widget]: widget/index.html#built-in-widgets
|
||||
/// [`Widget`]: widget/trait.Widget.html
|
||||
/// [`Element`]: struct.Element.html
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Element<'a, Message, Renderer> {
|
||||
pub(crate) widget: Box<dyn Widget<Message, Renderer> + 'a>,
|
||||
}
|
||||
|
@ -29,7 +29,7 @@
|
||||
//! [`Windowed`]: renderer/trait.Windowed.html
|
||||
//! [`UserInterface`]: struct.UserInterface.html
|
||||
#![deny(missing_docs)]
|
||||
//#![deny(missing_debug_implementations)]
|
||||
#![deny(missing_debug_implementations)]
|
||||
#![deny(unused_results)]
|
||||
#![deny(unsafe_code)]
|
||||
#![deny(rust_2018_idioms)]
|
||||
|
@ -5,6 +5,7 @@ use crate::{
|
||||
};
|
||||
|
||||
/// A renderer that does nothing.
|
||||
#[derive(Debug, Clone, Copy)]
|
||||
pub struct Null;
|
||||
|
||||
impl Renderer for Null {
|
||||
|
@ -10,6 +10,7 @@ use std::hash::Hasher;
|
||||
/// charge of using this type in your system in any way you want.
|
||||
///
|
||||
/// [`Layout`]: struct.Layout.html
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct UserInterface<'a, Message, Renderer> {
|
||||
hash: u64,
|
||||
root: Element<'a, Message, Renderer>,
|
||||
|
@ -12,7 +12,20 @@ use crate::{
|
||||
};
|
||||
use std::hash::Hash;
|
||||
|
||||
/// A generic widget that produces a message when clicked.
|
||||
/// A generic widget that produces a message when pressed.
|
||||
///
|
||||
/// ```
|
||||
/// # use iced_native::{button, Button};
|
||||
///
|
||||
/// enum Message {
|
||||
/// ButtonPressed,
|
||||
/// }
|
||||
///
|
||||
/// let mut state = button::State::new();
|
||||
/// let button = Button::new(&mut state, Text::new("Press me!"))
|
||||
/// .on_press(Message::ButtonPressed);
|
||||
/// ```
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Button<'a, Message, Renderer> {
|
||||
state: &'a mut State,
|
||||
content: Element<'a, Message, Renderer>,
|
||||
|
@ -25,6 +25,7 @@ use crate::{
|
||||
/// ```
|
||||
///
|
||||
/// 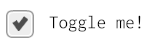
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Checkbox<Message> {
|
||||
is_checked: bool,
|
||||
on_toggle: Box<dyn Fn(bool) -> Message>,
|
||||
|
@ -12,6 +12,7 @@ use std::u32;
|
||||
/// A [`Column`] will try to fill the horizontal space of its container.
|
||||
///
|
||||
/// [`Column`]: struct.Column.html
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Column<'a, Message, Renderer> {
|
||||
spacing: u16,
|
||||
padding: u16,
|
||||
|
@ -10,7 +10,7 @@ use std::u32;
|
||||
/// An element decorating some content.
|
||||
///
|
||||
/// It is normally used for alignment purposes.
|
||||
#[allow(missing_docs)]
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Container<'a, Message, Renderer> {
|
||||
width: Length,
|
||||
height: Length,
|
||||
|
@ -33,6 +33,7 @@ use std::hash::Hash;
|
||||
/// ```
|
||||
///
|
||||
/// 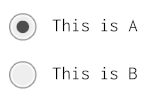
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Radio<Message> {
|
||||
is_selected: bool,
|
||||
on_click: Message,
|
||||
|
@ -12,7 +12,7 @@ use std::u32;
|
||||
/// A [`Row`] will try to fill the horizontal space of its container.
|
||||
///
|
||||
/// [`Row`]: struct.Row.html
|
||||
#[allow(missing_docs)]
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Row<'a, Message, Renderer> {
|
||||
spacing: u16,
|
||||
padding: u16,
|
||||
|
@ -10,6 +10,7 @@ use std::{f32, hash::Hash, u32};
|
||||
|
||||
/// A widget that can vertically display an infinite amount of content with a
|
||||
/// scrollbar.
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Scrollable<'a, Message, Renderer> {
|
||||
state: &'a mut State,
|
||||
height: Length,
|
||||
|
@ -35,6 +35,7 @@ use std::{hash::Hash, ops::RangeInclusive};
|
||||
/// ```
|
||||
///
|
||||
/// 
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct Slider<'a, Message> {
|
||||
state: &'a mut State,
|
||||
range: RangeInclusive<f32>,
|
||||
|
@ -30,6 +30,7 @@ use crate::{
|
||||
/// Message::TextInputChanged,
|
||||
/// );
|
||||
/// ```
|
||||
#[allow(missing_debug_implementations)]
|
||||
pub struct TextInput<'a, Message> {
|
||||
state: &'a mut State,
|
||||
placeholder: String,
|
||||
|
Loading…
Reference in New Issue
Block a user