diff --git a/tensorflow/lite/tools/benchmark/android/AndroidManifest.xml b/tensorflow/lite/tools/benchmark/android/AndroidManifest.xml
index 7cdca2885dd..ee46ff9bf02 100644
--- a/tensorflow/lite/tools/benchmark/android/AndroidManifest.xml
+++ b/tensorflow/lite/tools/benchmark/android/AndroidManifest.xml
@@ -21,22 +21,21 @@
-
+ android:minSdkVersion="23"
+ android:targetSdkVersion="23" />
-
+
-
+
diff --git a/tensorflow/lite/tools/benchmark/android/README.md b/tensorflow/lite/tools/benchmark/android/README.md
index 76463517db9..00fa01bb9bd 100644
--- a/tensorflow/lite/tools/benchmark/android/README.md
+++ b/tensorflow/lite/tools/benchmark/android/README.md
@@ -34,8 +34,10 @@ bazel build -c opt \
(2) Connect your phone. Install the benchmark APK to your phone with adb:
```
-adb install -r -d bazel-bin/tensorflow/lite/tools/benchmark/android/benchmark_model.apk
+adb install -r -d -g bazel-bin/tensorflow/lite/tools/benchmark/android/benchmark_model.apk
```
+Note: Make sure to install with "-g" option to grant the permission for reading
+extenal storage.
(3) Push the compute graph that you need to test.
@@ -50,9 +52,10 @@ and can be appended to the `args` string alongside the required `--graph` flag
args key).
```
-adb shell am start -S -n \
- org.tensorflow.lite.benchmark/org.tensorflow.lite.benchmark.BenchmarkModelActivity \
- --es args '"--graph=/data/local/tmp/mobilenet_quant_v1_224.tflite --num_threads=4"'
+adb shell am start -S \
+ -n org.tensorflow.lite.benchmark/.BenchmarkModelActivity \
+ --es args '"--graph=/data/local/tmp/mobilenet_quant_v1_224.tflite \
+ --num_threads=4"'
```
(5) The results will be available in Android's logcat, e.g.:
@@ -62,3 +65,69 @@ adb logcat | grep "Average inference"
... tflite : Average inference timings in us: Warmup: 91471, Init: 4108, Inference: 80660.1
```
+
+## To trace Tensorflow Lite internals including operator invocation
+
+The steps described here follows the method of
+https://developer.android.com/topic/performance/tracing/on-device. Refer to the
+page for more detailed information.
+
+(0)-(3) Follow the steps (0)-(3) of [build/install/run](#to_buildinstallrun)
+section.
+
+(4) Set up Quick Settings tile for System Tracing app on your device. Follow the
+[instruction]
+(https://developer.android.com/topic/performance/tracing/on-device#set-up-tile).
+The System Tracing tile will be added to the Quick Settings panel, which appears
+in next figure:
+
+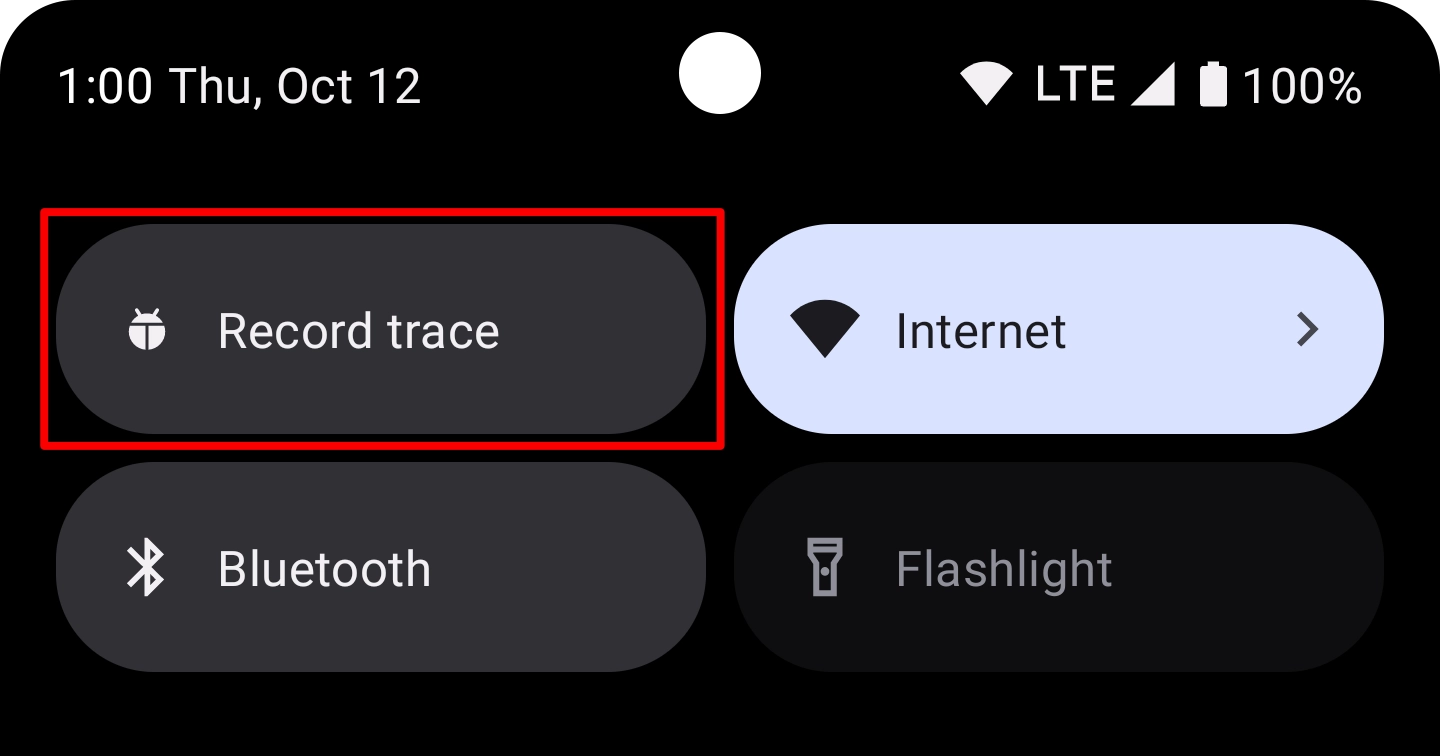{ width=40% }
+
+Optionally, you can set up other configurations for tracing from the app menu.
+Refer to the [guide]
+(https://developer.android.com/topic/performance/tracing/on-device#app-menu)
+for more information.
+
+(5) Tap the System Tracing tile, which has the label "Record trace". The tile
+becomes enabled, and a persistent notification appears to notify you that the
+system is now recording a trace, as shown in next figure:
+
+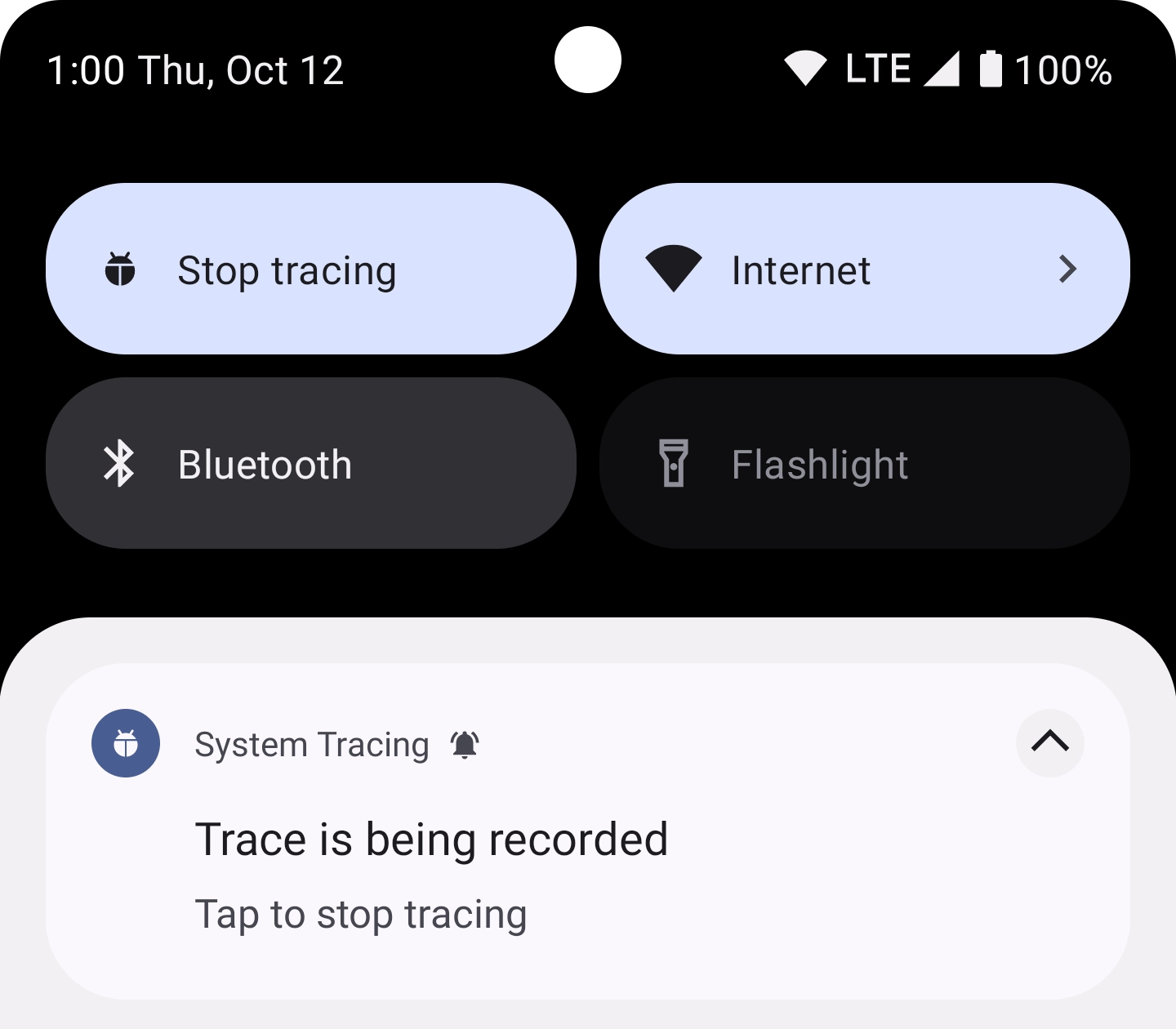{ width=40% }
+
+(6) Run the benchmark with platform tracing enabled.
+
+```
+adb shell am start -S \
+ -n org.tensorflow.lite.benchmark/.BenchmarkModelActivity \
+ --es args '"--graph=/data/local/tmp/mobilenet_quant_v1_224.tflite \
+ --num_threads=4 --enable_op_profiling=true --enable_platform_tracing=true"'
+```
+
+(7) Wait until the benchmark finishes. It can be checked from Android log
+messages, e.g.,
+
+```
+adb logcat | grep "Average inference"
+
+... tflite : Average inference timings in us: Warmup: 91471, Init: 4108, Inference: 80660.1
+```
+
+(8) Stop tracing by tapping either the System Tracing tile in the Quick Settings
+panel or on the System Tracing notification. The system displays a new
+notification that contains the message "Saving trace". When saving is complete,
+the system dismisses the notification and displays a third notification,
+confirming that your trace has been saved and that you're ready to share the
+system trace, as shown in next figure:
+
+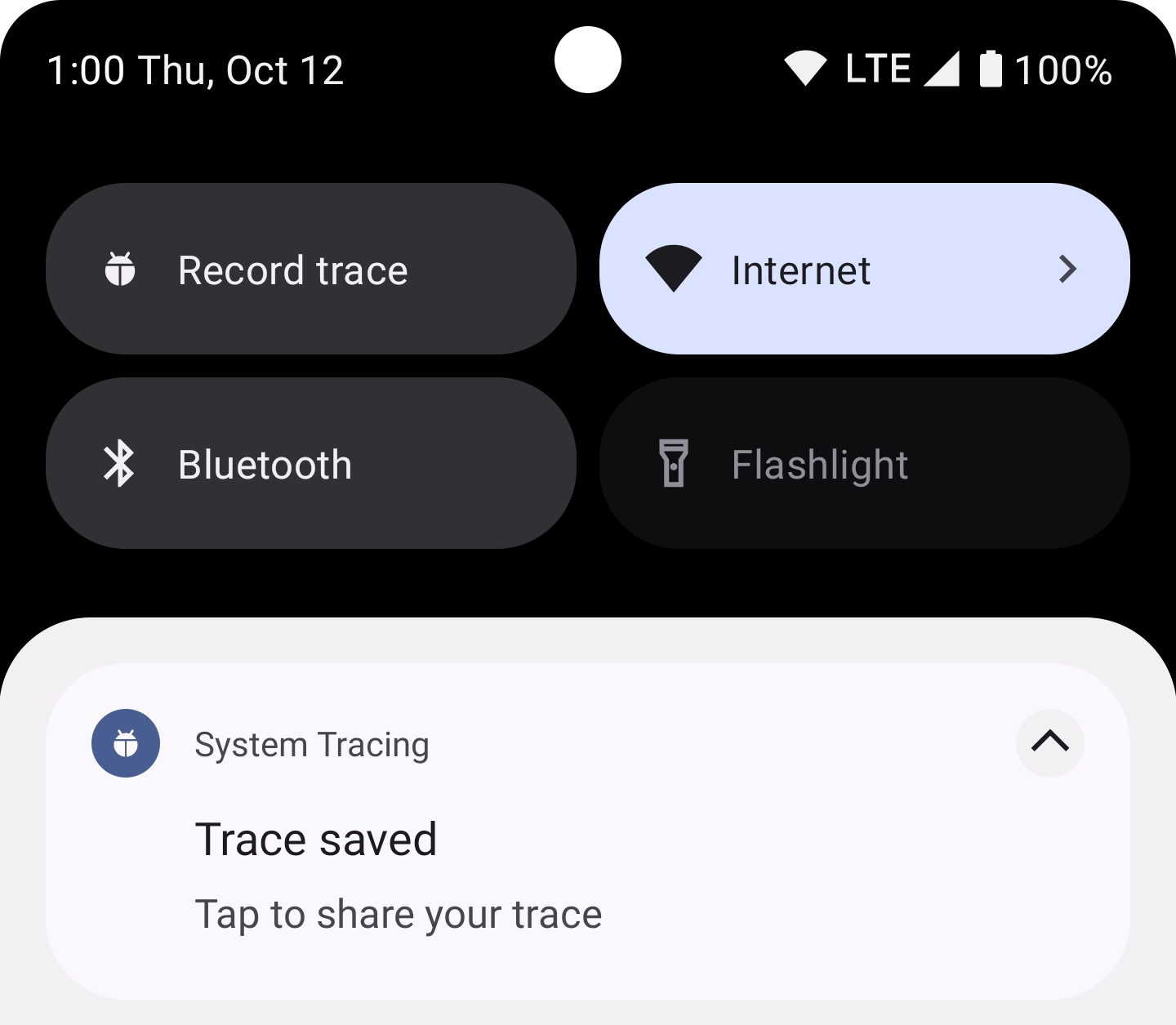{ width=40% }
+
+(9) [Share]
+(https://developer.android.com/topic/performance/tracing/on-device#share-trace)
+a trace file, [convert]
+(https://developer.android.com/topic/performance/tracing/on-device#converting_between_trace_formats)
+between tracing formats and [create]
+(https://developer.android.com/topic/performance/tracing/on-device#create-html-report)
+an HTML report.
+Note that, the catured tracing file format is either in Perfetto format or in
+Systrace format depending on the Android version of your device. Select the
+appropriate method to handle the generated file.
diff --git a/tensorflow/lite/tools/benchmark/android/src/org/tensorflow/lite/benchmark/BenchmarkModelActivity.java b/tensorflow/lite/tools/benchmark/android/src/org/tensorflow/lite/benchmark/BenchmarkModelActivity.java
index 12410adf3d6..6833d70931b 100644
--- a/tensorflow/lite/tools/benchmark/android/src/org/tensorflow/lite/benchmark/BenchmarkModelActivity.java
+++ b/tensorflow/lite/tools/benchmark/android/src/org/tensorflow/lite/benchmark/BenchmarkModelActivity.java
@@ -18,6 +18,7 @@ package org.tensorflow.lite.benchmark;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
+import android.os.Trace;
import android.util.Log;
/** Main {@code Activity} class for the benchmark app. */
@@ -37,7 +38,9 @@ public class BenchmarkModelActivity extends Activity {
String args = bundle.getString(ARGS_INTENT_KEY_0, bundle.getString(ARGS_INTENT_KEY_1));
Log.i(TAG, "Running TensorFlow Lite benchmark with args: " + args);
+ Trace.beginSection("TFLite Benchmark Model");
BenchmarkModel.run(args);
+ Trace.endSection();
finish();
}